Ayrshare Messaging API for Facebook, Instagram, and X/Twitter
In the world of digital marketing, apps and platforms are always seeking new tools to enhance their user engagement on their platforms. For years, Ayrshare has offered the industry leading social media API for scheduling posts, analytics, and managing comments.
Now we’re excited to introduce our first communications offering: the Ayrshare Messaging API – a comprehensive solution for managing real-time direct messages in Facebook Messenger, Instagram Messaging, and X/Twitter Direct Messaging.
Many of our clients have asked for the ability to communicate with their users with real-time messages. Some need messaging for support desk chat, others to send notifications like order updates, and most often to nurture sales leads. Now with the new Messaging API, you can manage your user’s direct messages to those who contact them across their preferred channels, allowing them to provide a seamless customer experience. Additionally, the convenience of using one API to manage messaging across different platforms streamlines your implementation and brings all the benefits of the Ayrshare platform.
This guide will walk you through the features, implementation, and potential applications of the Messaging API. If you want to get started quickly, you can also use the GUI version of messaging in the new Messages tab in the Ayrshare Dashboard.
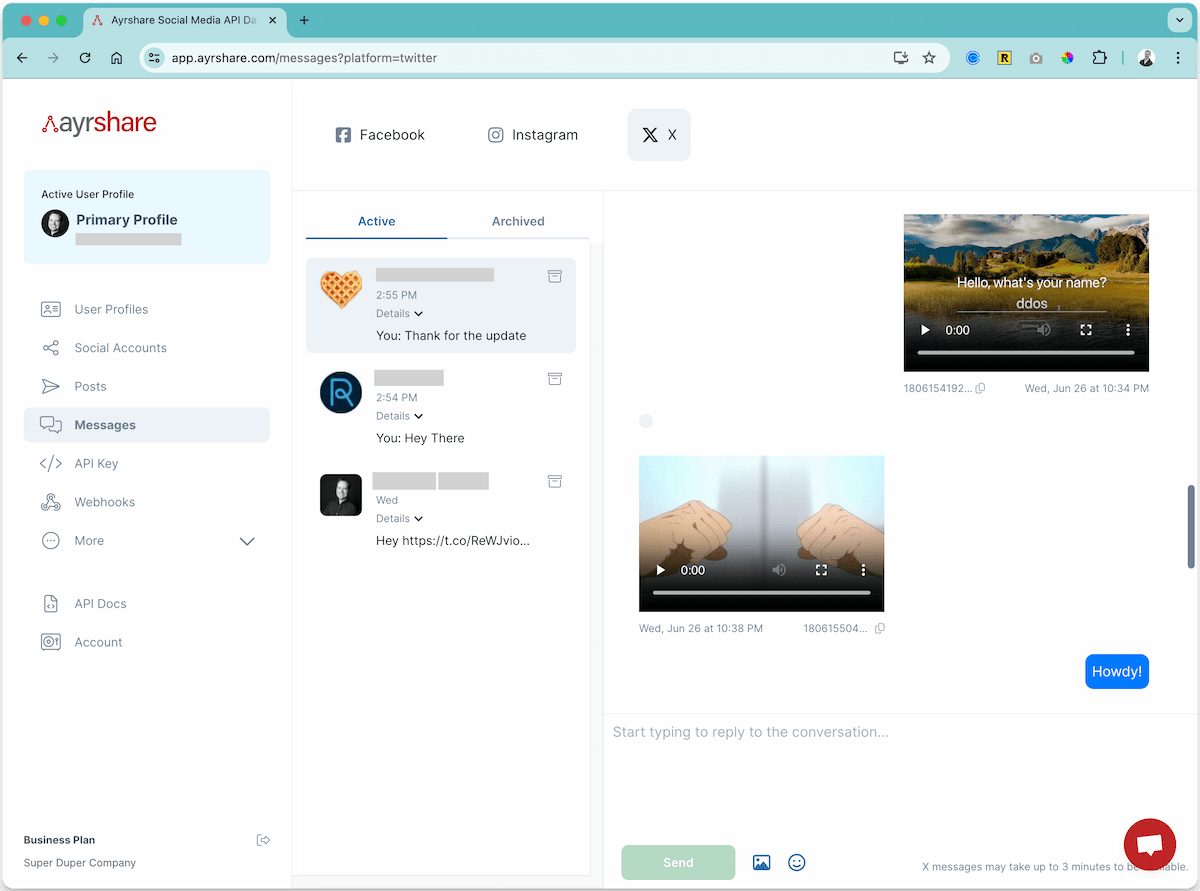
Using the Messaging API
The Messaging API has been designed to provide businesses with various messaging services, including sending and receiving messages via multiple social platforms. This includes posting messages (including text, images, videos, and emoji), getting messages, setting up auto responses, and registering webhooks to be notified of new messages.
1. Conversation Management
The Messaging API allows you to effortlessly retrieve and manage conversations and receive messages across multiple channels: Facebook Messenger, Instagram Messages, or X Direct Messages.
Retrieving Messages
Using the GET messages endpoint, you can fetch all messages for a specific platform. Here’s an example using cURL to retrieve Facebook Messenger messages using the GET messages API endpoint:
curl \
-H "Authorization: Bearer API_KEY" \
-X GET https://app.ayrshare.com/api/messages/facebook
The API returns comprehensive data, including:
- Message content
- Attachments (videos, images)
- Reactions (e.g., likes, hearts)
- Timestamps
- Sender and recipient information
Here is an example of the JSON returned:
{
"status": "success",
"messages": [
{
"senderId": "106638148652444",
"senderDetails": {
"name": "Ayrshare"
},
"conversationId": "t_10161117434308444",
"created": "2024-06-06T00:54:32.455Z",
"action": "sent",
"recipientId": "7101149746568444",
"id": "m_JH6o-yS83JoxWmQaLrmgSaHwGtfTgQ",
"message": "Howdy!",
"platform": "facebook",
"reactions": {
"7101149746568522": "😆". // Reaction by the customer on the Howdy! message
}
},
{
"senderId": "7101149746568444",
"senderDetails": {
"name": "John Smith",
"profileImage": "https://platform-lookaside.fbsbx.com/platform/profilepic/"
},
"conversationId": "t_10161117434308444",
"created": "2024-06-06T00:54:28.102Z",
"action": "received",
"recipientId": "106638148652329",
"id": "m_HGbotYJUmf4AzyPlJ-2uZqHwGtfTgQihX",
"message": "Look up!",
"platform": "facebook"
},
{
"senderId": "7101149746568444",
"senderDetails": {
"name": "John Smith",
"profileImage": "https://platform-lookaside.fbsbx.com/platform/profilepic/"
},
"conversationId": "t_10161117434308444",
"created": "2024-06-06T00:49:11.679Z",
"action": "received",
"recipientId": "106638148652444",
"id": "m_jXoYQIwTXaq2u06PG6Z8vaHwGtfTgQ",
"message": "How is the weather?",
"platform": "facebook"
}
],
"lastUpdated": "2024-06-09T21:46:04.233Z",
"nextUpdate": "2024-06-09T21:47:04.233Z"
}
Retrieving Conversations
Sometimes you need to get a list of the conversations for a platform. Just add on the query parameter conversationsOnly=true. Here is an example if getting the conversation for Instagram in Node.js:
const apiKey = 'API_KEY';
const url = 'https://app.ayrshare.com/api/messages/instagram?conversationsOnly=true';
const headers = {
'Authorization': `Bearer ${apiKey}`,
};
fetch(url, {
method: 'GET',
headers: headers,
})
.then(response => {
if (response.ok) {
return response.json();
} else {
throw new Error(`Request failed. Status code: ${response.status}`);
}
})
.then(data => {
console.log('Response:', data);
})
.catch(error => {
console.error('Error:', error.message);
});
And the JSON response of the conversations ids:
{
"status": "success",
"conversationIds": [
"t_10161117434308444",
"t_356759043857444"
],
"converstationsDetails": [
{
"id": "t_10161117434308444",
"participant": {
"name": "John Smith",
"id": "7101149746568444",
"picture": "https://platform-lookaside.fbsbx.com/platform/profilepic/"
},
"status": "active",
"watermark": 1717889607444
},
{
"id": "t_356759043857444",
"participant": {
"name": "Sara Johnson",
"id": "7365320280173444",
"picture": "https://platform-lookaside.fbsbx.com/platform/profilepic/"
},
"status": "active"
}
],
"lastUpdated": "2024-06-09T21:46:04.233Z",
"nextUpdate": "2024-06-09T21:47:04.233Z"
}
2. Multi-Media Message Sending
The POST messages endpoint enables you to send messages with various types of content, including SMS and MMS, to your recipients. This includes images, GIFs, videos, or emojis. Here’s a JavaScript example for sending a message with an image on Instagram Messenger using the POST messages API endpoint:
const apiKey = 'API_KEY';
const url = 'https://app.ayrshare.com/api/messages/instagram';
const data = {
message: "What's up!",
recipientId: '283j839222',
"mediaUrl": ["img.ayrshare.com/012/gb.jpg"]
};
const headers = {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json',
};
fetch(url, {
method: 'POST',
headers: headers,
body: JSON.stringify(data),
})
.then(response => response.json())
.then(data => {
console.log('Response:', data);
})
.catch(error => {
console.error('Error:', error);
});
3. Automated Responses
One of the more powerful features is the ability to set up automatic responses. This ensures that your users always receive a prompt acknowledgment, even when you’re not immediately available. For example, the API can also be used to send replies when you are not online, or one-time passcodes for secure communications. Here’s how to set it up using Python and the set auto response API endpoint to response with “Howdy!”:
import json
import requests
url = 'https://app.ayrshare.com/api/messages/autoresponse'
headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer API_KEY'
}
data = {
'autoResponseActive': True,
'autoResponseWaitSeconds': 30,
'autoResponseMessage': 'Howdy!'
}
response = requests.post(url, headers=headers, data=json.dumps(data))
try:
response.raise_for_status()
data = response.json()
print(data)
except requests.exceptions.RequestException as e:
print('Error:', e)
4. Real-Time Notifications with Webhooks
You can stay on top of your conversations across the social channels with real-time webhooks. For Facebook and Instagram, you can register a Messages Action Webhook to receive notifications for new messages, read receipts, or reactions, such as thumbs ups on a message.
Here’s an example of the JSON payload you’ll receive when a message is read:
{
"action": "messages",
"code": 200,
"conversationId": "t_10161117434308936",
"created": "2024-06-08T23:33:30Z",
"hookId": "CviPBMXEy3cdJnK0EESd",
"mediaUrls": [],
"platform": "facebook",
"read": 1717889607802,
"readerDetails": {
"name": "John Smith",
"id": "7101149746568444",
"picture": "https://platform-lookaside.fbsbx.com/platform/profilepic"
},
"recipientId": "106638148652329",
"refId": "9abf1426d6ce9122ef11c8932",
"scheduleDate": "2024-06-08T23:33:30Z",
"senderId": "7101149746568522",
"subAction": "messageRead",
"timeStamp": 1717889610,
"title": "Primary Profile",
"type": "read",
"url": "https://mysite.com/webhook"
}
Implementing the Ayrshare Messages API
Like all Ayrshare APIs, you can quickly get started with the Ayrshare Messaging API:
- Ensure that you have a subscription to the Ayrshare Business Plan. If you’re not already a Business Plan subscriber, please contact us.
- Enable messaging in your Account settings within the Dashboard. Please note messaging is a paid add-on.
- Head to the User Profiles page and click the “Messaging active” checkbox to activate messaging for each User Profile. Users will need to relink Facebook, Instagram, and X to activate messaging.
- You’re now set to start using the Messaging API. Now your users can communicate with customers through their preferred channels.
You can also programmatically activate messaging for a User Profile creation by adding messagingActive: true with the profiles endpoint:
const API_KEY = "API_KEY";
fetch("https://app.ayrshare.com/api/profiles", {
method: "POST",
headers: {
"Content-Type": "application/json",
"Authorization": `Bearer ${API_KEY}`
},
body: JSON.stringify({
title: "ACME Profile", // required
messagingActive: true
}),
})
.then((res) => res.json())
.then((json) => console.log(json))
.catch(console.error);
Unlocking New Possibilities
The Ayrshare Messaging API opens up possibilities for new integrations into your platform:
- Enhanced Customer Support: Implement a chatbot that handles initial inquiries and seamlessly transfers to human agents when needed.
- Personalized Marketing: Send targeted product recommendations based on user interactions and preferences.
- Automated Notifications: Keep users informed about order status, account updates, or upcoming events.
- Lead Nurturing: Develop a messaging funnel that guides potential customers through the sales process.
- Community Management: Engage with your audience across multiple platforms from a single interface.
- Personalized Experiences: Deliver tailored interactions and content, such as alerts, reminders, and notifications, to enhance user engagement.
The Ayrshare Messaging API represents a leap forward in cross-platform messaging capabilities. By providing a unified interface for Facebook Messenger, Instagram Messaging, and X/Twitter Direct Messaging, it allows businesses to create more engaging, responsive, and personalized communication strategies.
We are very excited about the new Messaging API capabilities of Ayrshare and are here to help as you integrate. For more information on all Ayrshare products, detailed documentation, and support, visit the Ayrshare website.