Best Practices for API Design
Tips for Creating Well-Structured and Maintainable APIs
At Ayrshare, we continue to see Application Programming Interfaces (APIs) adopted as the backbone of seamless communication between different software systems. And just like a good (dad) joke, an API should be easy to understand and leave a lasting impression. You wouldn’t want developers scratching their heads and saying, “I don’t get it!” – translation “I’m outta-here.”
A well-designed API ensures a great developer experience, which leads to happy users and happy clients.
In this article, we’ll discuss some tips and guidelines for designing APIs with some examples from Ayrshare’s own REST API endpoints.
Understand Your Developer Audience
Before writing a single line of code, it’s imperative to know who will be using your API. Are they seasoned developers or newcomers? Understanding your target audience influences decisions on complexity, documentation style, and the level of abstraction. Think of your own personal use-case – what are you looking for when you read the docs for a company’s API, e.g. Stripe.
At Ayrshare, we designed our API with both simplicity and flexibility in mind, creating something that we like using ourselves. Whether you’re a startup developer looking to integrate social media posting quickly or an enterprise needing advanced features like analytics and user management, our API caters to a wide range of skill levels and requirements.
Establish Consistency and Standards
Consistency is key in API design. Do you want a jumble of different standards, for example some API response use camel case (camelCase) and others snake case (snake_case)? And for the same data different field names are used like age and user_age? Yuck.
By having a consistent standard, you establish a sensible contract with your users and reduce their learning curve, frustration, and hopefully prevents errors.
When establishing API consistency and standards focus on:
- HTTP Methods: Adhere to RESTful principles by using HTTP methods appropriately:
GET
for retrieving resourcesPOST
for creating resourcesPUT
orPATCH
for updating resourcesDELETE
for deleting resources
- Response Field Names: If one endpoint response is
userId
, don’t useid
in another endpoint response if they are the same value. - Data Formats: Stick to standard data formats like Zulu time to ensure compatibility and universality.
- Endpoint URL Structure: A lot of API philosophies exist on how to structure the endpoint URL, for example
GET
https://api.ayrshare.com/api/analytics
. In this example the action isGET
and the type isanalytics
. It really doesn’t matter if this is your style vsGET
https://api.ayrshare.com/api/getAnalytics
l The important point is to be consistent on all the endpoints. - HTTP Response Codes: The HTTP response code is the first indication to the API user the call was successful or if a problem occurred. For example, a response in the 200 range means everything is good. A response 400 or above means here was an issue.
Examples:
Creating a Post: To create a new social media post, Ayrshare uses the POST
method with the /post
endpoint:
POST https://api.ayrshare.com/api/post
Request Body:
{
"post": "Hello World!",
"platforms": ["facebook"]
}
Response Body:
{
"status": "success",
"errors": [],
"postIds": [
{
"status": "success",
"id": "1835773643315433893",
"postUrl": "https://www.facebook.com/33892",
"platform": "facebook"
}
],
"id": "G5y3PRI7bzLq1GmV5Xaa",
"refId": "9abf1426d6ce9122ef11c72bd62e59807c5cc092",
"post": "A beautiful day",
"validate": true
}
Consider always returning a status
, such as "error"
or "success"
and be sure it matches with the HTTP response code.
Retrieving a Post: To retrieve details of a specific post, use the GET
method with the /post/{id}
endpoint:
GET https://app.ayrshare.com/api/post/1234567890
This consistent use of HTTP methods and endpoint structures makes the API intuitive and easy to use.
Design with Scalability in Mind
As your API grows, it should handle increased load without performance degradation.
- Statelessness: Design your API to be stateless. Each request should contain all the information needed to process it, enabling better scalability. Each Ayrshare API request includes all the necessary information, such as the API key and parameters, ensuring that the server doesn’t need to retain session information between requests.
- Efficient Resource Management: Optimize your endpoints to handle multiple operations efficiently. If everything goes well, you’ll be getting a lot of API calls and need to be able to support them all. Consider a serverless infrastructure – such as Supabase or Firebase – to automatically handle increased load, but be warned auto scaling can get expensive, so be sure to set budget limits..
- Load Balancing and Rate Limiting: Implement strategies to distribute load and prevent abuse. To maintain optimal performance and prevent abuse, Ayrshare implements fair-use rate limiting, detailed in our documentation, to ensure fair usage among all clients. Return the
429
HTTP response code if there was a rate limit error. - Bulk Operations: Ayrshare allows you to send a post to multiple social media platforms in a single request, reducing the number of API calls. This is good for your user and your system.
{
"post": "Exciting news! Our app just got better.",
"platforms": ["twitter", "facebook", "linkedin", "instagram"]
}
Implement a Clear Deprecation Policy
As your API evolves, certain features or endpoints may become obsolete. Implementing a clear deprecation policy ensures that changes to your API don’t break existing integrations and gives developers ample time to adapt.
- Advance Notice: Communicate upcoming changes well in advance. This can be done through email notifications, dashboard alerts, or dedicated announcements.
- Documentation Updates: Clearly mark deprecated features in your documentation, including the deprecation date and the planned removal date.
- Grace Periods: Provide reasonable time frames during which both the old and new features are supported, allowing developers to transition smoothly.
- Alternative Solutions: Whenever possible, suggest alternative endpoints or methods that developers can use in place of the deprecated features.
When we plan to deprecate an endpoint or feature at Ayrshare, we announce it through:
- Email Communications: Subscribers receive information in the monthly newsletter about the changes, including timelines.
- Documentation Updates: Deprecated features are clearly indicated in our documentation, with guidance on alternatives. This is in the upcoming API changes page.
- Grace Period: We ensure that deprecated features remain functional for a significant period, typically several months, to give developers ample time to update their applications. Sometimes the social networks only give 60 days, but in those cases we either try to find an alternative or let our users know right away.
- Support During Transition: Our support team is available to assist developers in migrating to new features or endpoints, ensuring minimal disruption to their services.
By focusing on a robust deprecation policy, Ayrshare maintains a stable and reliable API environment, even as we introduce new features and improvements.
Prioritize Security Measures
Protecting data and ensuring secure communication is non-negotiable. NON-NEGOTIABLE!
Authentication and Authorization: Implement robust authentication mechanisms like API keys or OAuth 2.0. Ayrshare uses Bearer API keys for authentication. Include your API key in the Authorization
header:
Authorization: Bearer YOUR_API_KEY
HTTPS Everywhere: Enforce HTTPS to encrypt data in transit. Ayrshare endpoints require HTTPS, ensuring all data transmitted is encrypted.
Security Testing: Perform regular PEN (Penetration Testing) on your APIs. If you don’t try to break them, someone else will.
Provide Clear and Comprehensive Documentation
Good documentation is as important as the API itself.
API Reference: Detailed information about each endpoint, parameters, request and response examples is essential. A lot of info is ok as long as it is organized. Here is an example of our post endpoint:
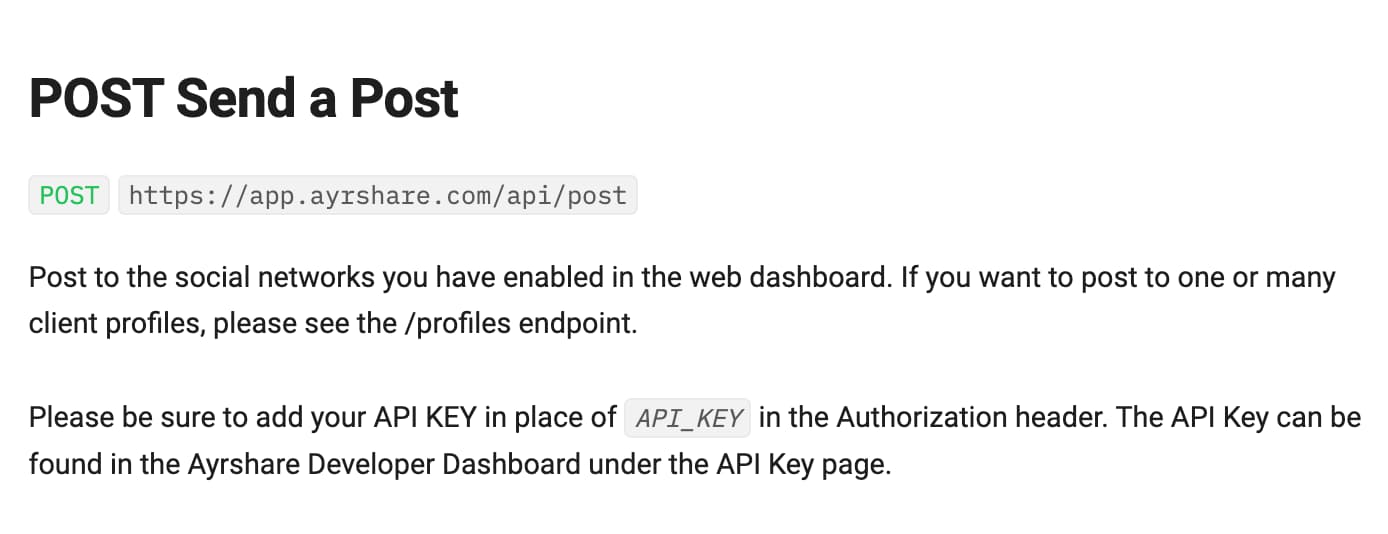
Tutorials and Guides: Provide step-by-step guides for common tasks to help developers get started quickly. A written tutorial is great, but a video tutorial will make you shine! For example here is our Make integration video guide:
Code Samples: Examples in multiple programming languages. AI today is great for translating from one programing language to another, but people still like to see examples in their preferred language and making it pretty also helps. For example:
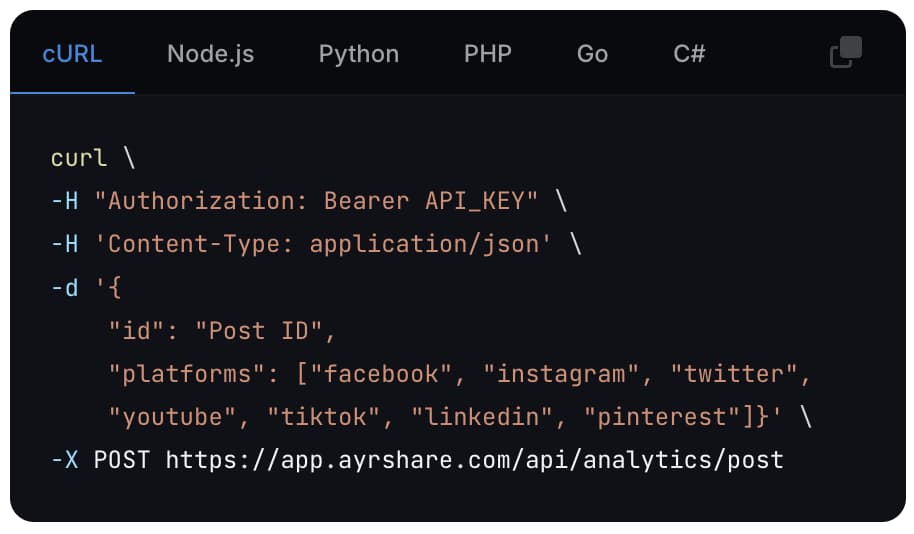
Implement Robust Error Handling
Clear and consistent error handling aids in debugging and improves developer experience.
- Standard HTTP Status Codes: Use appropriate status codes to indicate the result of an API call. Please see above where this is discussed.
- Descriptive Error Messages: Provide meaningful error messages and codes that help identify and resolve issues.
- Error Object Structure: Maintain a consistent structure for error responses.
For example, here is the error for deleting a post where the post id sent was not found:
HTTP/1.1 400 Bad Request
Content-Type: application/json
{
"action": "delete",
"status": "error",
"code": 114,
"message": "Delete id not found.",
"id": "IdpUOyihLEpIx0d0N9mI"
}
Facilitate Easy Testing and Monitoring
Ensure your API is reliable and performs well under various conditions.
- Automated Testing: Implement unit and integration tests to catch issues early. We are a big fan of Hurl to automate our API testing and recommend it to our users.
- Monitoring Tools: Use monitoring services to track API performance and uptime. We use this to drive our internal monitoring and to also for sending out status updates to clients.
- Logging: Keep detailed logs for auditing and troubleshooting purposes. This is critical for customer support. A good logging system will make your life easier, trust us.
- Internal Tooling: As your business grows, you’ll find a need for tooling to support your API users. Our favorite platform to build internally is Retool.
- External Testing: Finally your users need to be able to test your API. We recommend Postman and even provide a Postman JSON file that has all our endpoint predefined – makes calls really easy.
At Ayrshare, we understand the importance of robust API design. Our Social Media API is built with these best practices in mind, ensuring seamless integration and a superior developer experience.